I have a client who is using the testimonial carousel in Elementor. There are different text lengths in each content box resulting in the slider being way too big in mobile.
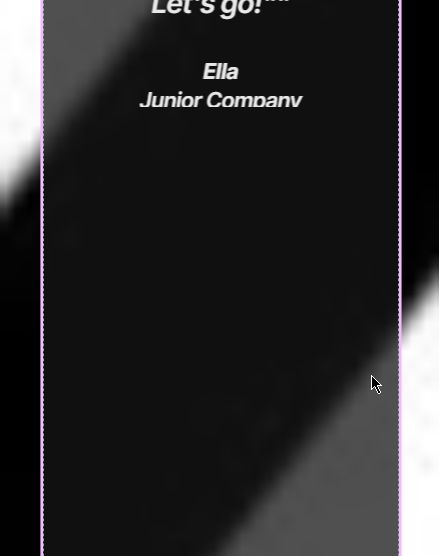
We needed a way to make the testimonial box auto resize to the height of the testimonial.
I notice the setup is like this:
<div class="elementor-swiper">
<div class="swiper-slide swiper-slide-active"><div class="elementor-testimonial">This is my slider text</div></div>
<div class="swiper-slide"><div class="elementor-testimonial">This is my slider text</div></div>
<div class="swiper-slide"><div class="elementor-testimonial">This is my slider text</div></div>
<div class="swiper-slide"><div class="elementor-testimonial">This is my slider text</div></div>
</div>
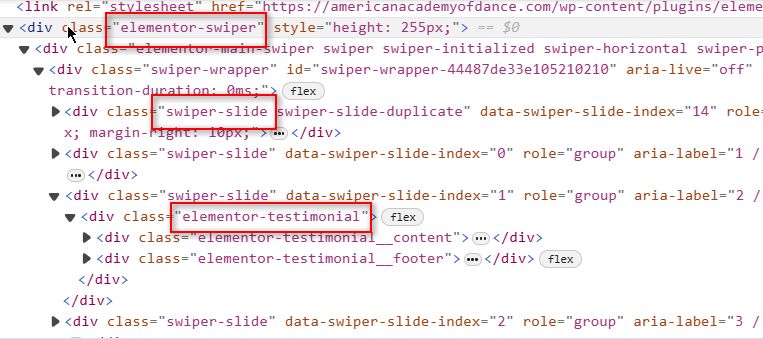
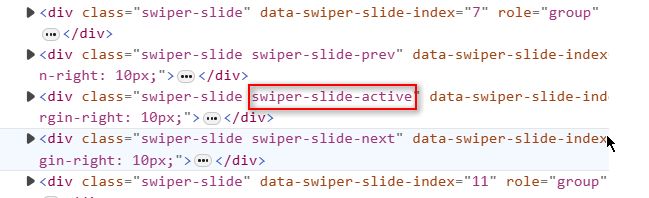
elementor-swiper
is the wrapper of the entire carousel
swiper-slide
wraps each slide
swiper-slide-active
is the active testimonial and switches automatically with JavaScript
elementor-testimonial
wraps the actual text, image, name and byline
We need a solution that:
- Detects when swiper-slide-active has switched.
- Measures the height of elementor-testimonial
- elementor-swiper and swiper-slide dynamically adjusts to the height of elementor-testimonial
The following solution will dynamically change elementor’s testimonial carousel height to the height of the testimonial:
jQuery(document).ready(function($) {
// Function to update the height of the swiper container and slides
function updateSwiperHeight() {
var activeSlide = $('.swiper-slide-active');
var testimonialHeight = activeSlide.find('.elementor-testimonial').outerHeight();
var newHeight = testimonialHeight + 50; // Add 50px to the height
$('.elementor-swiper, .swiper-slide').height(newHeight);
}
// Initial call to set the height based on the active slide
updateSwiperHeight();
// Observer to detect class changes on swiper-slide elements
var observer = new MutationObserver(function(mutations) {
mutations.forEach(function(mutation) {
if ($(mutation.target).hasClass('swiper-slide-active')) {
updateSwiperHeight();
}
});
});
// Observe each swiper-slide element for class attribute changes
$('.swiper-slide').each(function() {
observer.observe(this, {
attributes: true,
attributeFilter: ['class']
});
});
});
Explanation:
- Find the active slide:
var activeSlide = $('.swiper-slide-active');
- Measure the height: var testimonialHeight = activeSlide.find('.elementor-testimonial').outerHeight();
- Add 50px to the height: var newHeight = testimonialHeight + 50;
- Set the new height: $('.elementor-swiper, .swiper-slide').height(newHeight);
- Create the observer: var observer = new MutationObserver(function(mutations) {…});
- Check each mutation: mutations.forEach(function(mutation) {…});
- Update height if active slide changed: if ($(mutation.target).hasClass('swiper-slide-active')) { updateSwiperHeight(); }
This script dynamically adjusts the height of the elementor-swiper
container and all swiper-slide
elements whenever the active slide changes. It measures the height of the elementor-testimonial
element within the active slide, adds 50px to this height, and sets this new height on the container and slides, ensuring a smooth transition effect.